How to use
Primary info about UI Healthbars
First of all, you need to know, that there should be two objects on a scene to start using Healthbars Kit.
First one is an empty object with UIHealthbars component:
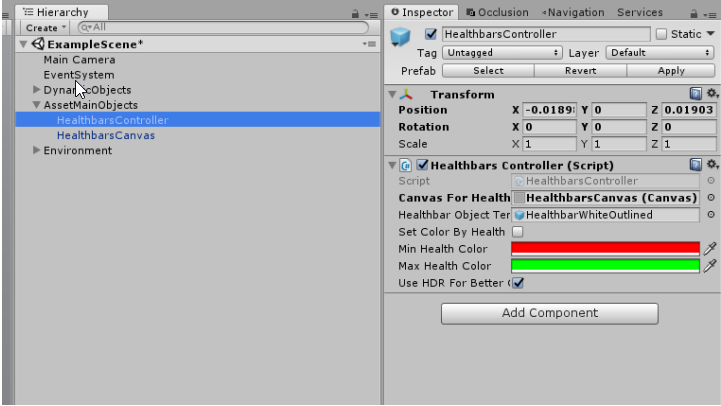
Second one needed object is the Canvas to draw healthbars. You can use your own or use our HealthbarsCanvas object from the Example scene. Its settings are very simple (similar to default Unity Canvas settings):
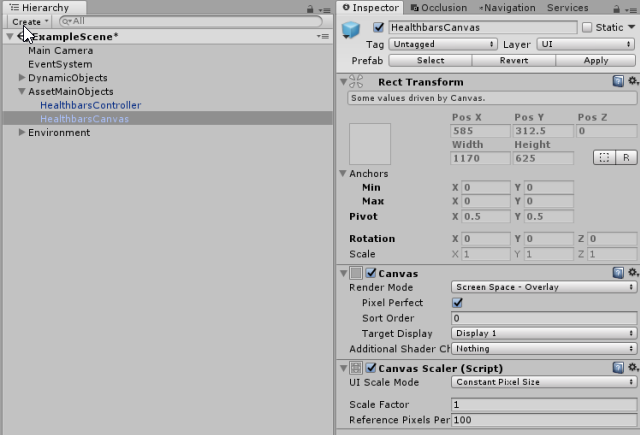
After this, you need drag your canvas to UIHealthbars component Healthbars Canvas field.
Now all ready to adding healthbars to your units. There are two ways how it can be done.
Setup using ready-made component
If your units actually have not any components, which are responsible for health, unit damage and unit death, you can use ready Damageable component from the Healthbars Kit. It has health and max health parameters and Take Damage method, which allows to deal damage to the unit. Also it has Die method, which responsible to unit death and actions which will be started on this event. You can extend this component to your needs or create inherited class with more parameters.
To use this Damageable, simple add this component to any of your units (and next you can make Prefab from this unit):
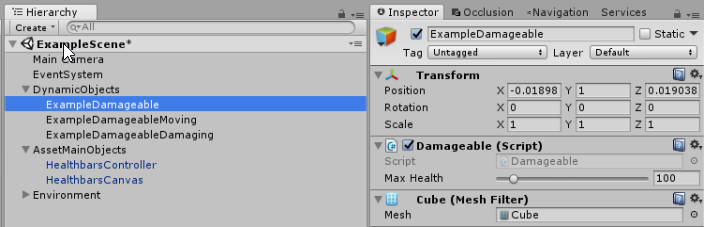
Now you can enter play mode and you should see healthbar above this unit:
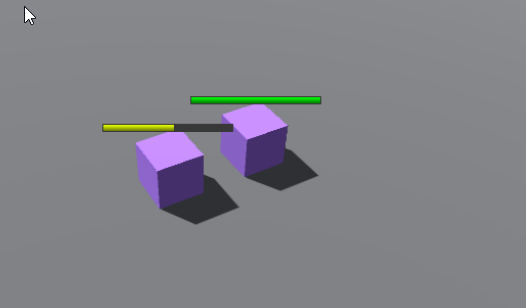
Setup from code
This is alternative setup method.
If you already have component with unit health and take damage methods, you should add new code to it. Btw, You can find and copy all code, shown in this guide, in the Damageable script.
Firstly, add event to the beginning of your script:
public event UIHealthbars.HealthChangedAction HealthWasChanged;
Note
Don’t forget to add using reference to the InsaneSystems.HealthbarsKit.UI.
Next, you need to add AddHealthbar and OnHealthChanged methods:
void Start()
{
var healthBar = UIHealthbars.AddHealthbar(gameObject, maxHealth);
// Setting up event to connect the Healthbar with this Damageable.
// Now every time when it will take damage, Healthbar will be updated.
HealthWasChanged += healthBar.OnHealthChanged;
OnHealthChanged();
}
void OnHealthChanged() => HealthWasChanged?.Invoke(health);
Finally, add call of the OnHealthChanged method in place, where you deal damage to your unit:
public void TakeDamage(float damage)
{
health = Mathf.Clamp(health - damage, 0, maxHealth);
OnHealthChanged();
if (health == 0)
Die();
}
Note
You need also add OnHealthChanged in healing part of your code, if you have it.
Done!